Software Implementation Event Assignment - Robot Coding
MAIN OBJECTIVE OF THE ASSESSMENT
This assignment is used to assess your problem-solving skills and your ability to implement a solution for a given problem in Java that would control your finch robot. Even though this assignment is assessed individually you may still discuss your work with the other members in your L1 project group. The assessment will be done during a whole day event called Robot Olympics during which you will be asked to demonstrate your Java finch program.
DESCRIPTION OF THE ASSESSMENT
Each member of the group will be expected to produce a program in Java for just one of the problems from the list of options provided below. Please note that each member of your group should choose a different problem. In other words, two members of a group CANNOT submit programs addressing the same problem.
Option 1: Following a Light
The purpose of this program is to make your finch robot follow a light source, such as a flashlight. To make your finch robot start, you should direct a light beam at the finch. This makes the finch to first turn its LED light colour into green (your choice of shade of green) and then to start moving towards the light source making a pleasant and not too loud sound as long as it is moving; we can call it 'on-the-move' sound. You should experiment with the sounds to find the sound you find suitable for this task. The finch should stop moving if
1. the light source is switched off or
2. it has encountered an obstacle.
Every time the finch stops moving it should change its colour to red and should stop making 'on-the-move' sound. The program should stop if the light remains switched off for longer than 5 seconds or, in the case of an obstacle, if the light beam does not move away from the obstacle within 5 seconds. However, once there are right conditions again (i.e., the light is on and/or it is no longer pointing at the obstacle) the finch will change its LED light colour back to green and resume moving and making its 'on-the-move' sound. The program should store the sequence of actions (including stops) executed by the robot in order to follow the light. Before the program ends, it should display the sequence of actions and the total count for the most frequent action. One can consider 5 basic actions/primitives: forward, left, right, stop (with speed, angle, time, etc. just being parameters associated to these actions). For example, if the 'executed' robot sequence was: forward (speed1), stop (), forward (speed2), left (angle1), right (angle2), forward (speed3), stop () then the most frequent action in the above example was forward.
Option 2: Drawing
The purpose of this program is to make your finch robot draw a shape. It should be able to draw rectangles and triangles. The finch should be placed on a large piece of paper on a flat surface with a pencil inserted in its tail. When the program starts the user is asked to enter a shape for the finch to draw or to quit the program using one of the following command:
- R (for a rectangle)
- T (for a triangle)
- Q (to stop)
If 'R' is entered the user will be asked to enter the rectangle size determined by its width and height. The rectangle width and height should be integer numbers (min value 40cm and max value 100cm). If the entered values are outside the range the program should inform the user about the type of the error and asked them to re-enter the values.
If 'T' is entered the user will be asked to enter the length of its three sides. The side values should be integers where no side is smaller than 25cm and no side is longer than 95cm. If the entered values are outside the range the program should inform the user about the type of the error and ask them to re-enter the values. Once the valid values are entered the program should check whether the entered numbers could form a triangle, i.e. the lengths of any two sides of a triangle must be greater than the length of the third side. Please note that before attempting to draw the triangle your program should determine triangle angles. Once the valid values are entered the finch should start moving so that its tail draws the desired shape. When the drawing of a shape is completed the robots should stop and beep once. The program should carry on prompting the user to select a shape to draw until the user enters Q. When Q is entered the robot should beep twice, display all drawn shapes and given size (e.g., Rectangle 47, 60), and for triangles all three calculated angles, in the order they were drawn and then stop all activity.
Option 3: Remote Navigation
The purpose of this program is to navigate the finch robot using commands entered from the keyboard. The finch should be able to move on a flat surface clear of any obstacles. The available navigation commands are:
- F (for forward movement)
- R (for right turn)
- L (for left turn)
- B (for backtracking the movement)
- Q (stop the program)
Each of the issued commands should be entered in a separate line. The format of the commands differs depending on what is controlled by the command. If an invalid command is entered the program should issue an appropriate message and ask the user to enter a valid command. When command 'Q' is issued the finch should stop.
The command 'F' is followed by two integer values separated by a single space. The first values determine the duration of the move in seconds and the second value sets the speed of the finch. No forward movement should last more than 6 seconds. The valid speed values are from -100 (full speed backwards) to 100 (full speed forward). For example, F 4 100 should be interpreted as a command to move the finch forward for 4 seconds at the speed of 100.
Commands 'R' and 'L' are followed by two integer values separated by single spaces. The first value sets the duration of the move in seconds and the second value sets the speed of the speed of the finch after the turn is made. No right or left movement should last more than 6 seconds. Assume that the right/left turn is always orthogonal to the current course.
Your program should make appropriate checks ensuring that the input values are within the valid range. You should work out the valid ranges for speed of each of the wheels for the command to have the desired effect on the right or the left turn.
Command 'B' is followed by an integer value that determines how many commands the finch needs to retrace. Of course, the number of backtrack moves cannot exceed the number of the move commands the finch has completed so far.
Option 4: Zigzag Path
The purpose of this program is to make your finch robot move in a regular zigzag fashion. In this problem we will assume that a zigzag path consists of lines of equal length that are orthogonal to each other (see http://en.wikipedia.org/wiki/Zigzag ). At the beginning of the program the user will be asked to enter the length of a zigzag section in centimetres and the number of zigzag sections (all sections are of the same length). For example, the zigzag in the illustration below consists of 9 sections. The length of a zigzag section should be at least 35 centimetres and no more than 65 centimetres. The number of zigzag sections should not exceed 8. Your program should check that valid values are entered and should generate appropriate error messages when this is not the case and would ask the user to re-enter the values within the valid range. The finch robot should move at a constant speed of 50. To test the program you can insert a pencil in the finch tail and let the finch travel on the piece of paper. Make sure to keep the length of the zigzag short unless you have very large piece of paper.
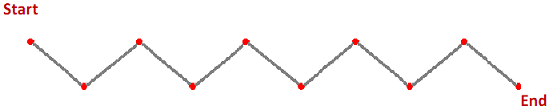
Once the length and the number of zigzag sections are provided the finch should turn its LED light into purple colour (your choice of purple shade) and start moving forwards at speed 50 until it moves for the given zigzag section length. As long it is moving it should be making a pleasant and not too loud sound (call it tune1). The finch should then briefly stop, turn orthogonally to the path travelled and start moving in the new direction for the length of zigzag line. As it is traversing the second section, its LED light should change to orange colour (again, your choice of orange shade) and will be producing a different sound (call it tune2). At the third section it should reverse its LED colour back to purple and the sound to tune1, used in the first section of the zigzag. In the fourth section the finch will go back to orange and tune2, and so on. Once the finch completes traversing the set number of zigzag sections it should turn and re-trace its movement until it reaches the original starting position (including the accompanying LED light colours and sounds as the first time it traversed that section). When the finch robot reaches the initial starting position it should stop and turn off the LED light and the sound. Before program stops, it should display the length of the traversed path (start to the end position of the zigzag, but not including travelling back) and the distance travelled (air distance) from Start to End of zigzag.
Option 5: Following an Object
The purpose of this program is to make your finch robot follow an object. To make your finch robot start, you should place an object in front of it and then tap the finch once encouraging it to start following the object. As long as the object does not move the finch will have its beak in red colour. Once the object starts moving the finch will turn the LED light to green colour and start moving behind the object. As long as the finch is moving it should be making not too loud buzzing sound. Every time the object stops moving the finch should also stop and it should turn the LED light from green to red and stop making the buzzing noise. Every time the object starts moving again, the finch should change the LED light back to green colour and resume the buzzing noise and start following the object again. The program stops once the object stops and the finch is tapped twice. The program should store the sequence of actions (including stops) executed by the robot in order to follow the object. Before the program ends, it should display the sequence of actions and the total count for the most frequent action. One can consider 5 basic actions/primitives: forward, left, right, stop (with speed, angle, time, etc. just being parameters associated to these actions). For example, if the 'executed' robot sequence was: forward (speed1), stop (), forward (speed2), left (angle1), right (angle2), forward (speed3), stop () then the most frequent action in the above example was forward.
Option 6: Dancing Finch
The purpose of this program is to make your finch robot perform a funny dance routine in which steps consist of only forward and backwards moves. The dance is determined by the user input. However, we will make this problem slightly more interesting by using different number systems. So your program will also need to serve as a number convertor. We will be using binary, decimal and hexadecimal number systems. See below. When the program starts it asks the user to enter a hexadecimal number of length 2 (e.g., 1F). The smallest number the user should enter is 90 (decimal equivalent 144) and the largest is FF (decimal equivalent 255). The program then converts this hexadecimal number into the decimal and the binary equivalents. For example, if the user enters A1 the decimal equivalent is 161 and the binary equivalent is 10100001. Once the conversion is done these numbers will be used for directing the dance. The speed of the finch will be determined by the decimal equivalent of the given number and the finch moves by the binary equivalent of the hexadecimal number. The speed of the finch robot will be set to the remainder of the division of the decimal number with 80. However, if the remainder is smaller than 40 then the speed should be set to reminder + 30. Before it starts moving the program should display the hexadecimal value given, the decimal and the binary equivalents and the speed at which the finch robot will be moving (e.g., A1, 161, 10100001, speed = 31) . The finch LED light should be set to the colour of your choice and once the finch starts moving it should produce a pleasant noise.
The moving will be as follows: reading the binary number equivalent from right to left one digit at a time the finch will move forward for 6 seconds when the digit is equal to 1 and backwards for 5 seconds when the digit is equal to zero. When the string of digits ends and the finch completes its last move it should switch off the noise and the LED light.
FORMAT OF THE ASSESSMENT
This assignment is individually assessed. After the submission is made you will have to demonstrate you Finch Robot program. The assessment will be done during a whole day event called Robot Olympics which will take place on Wednesday 15th march 2017. This event will be used to assess both Assignment 3 and Assignment 4. Please note that precise Finch movements may not be always possible. However, as long as the movements are coded correctly your work won't be penalised.
SUBMISSION INSTRUCTIONS
You must submit a single zip file consisting of all JAVA files of your Finch Robot program and your Report (which should be either a pdf or an MS Word document file). Your submission must be uploaded via Blackboard by 23:59GMT, Friday 10th March 2017 a single zip file. Your student ID number must be used for the file name (e.g. 0123456.zip). Your Report should not exceeding 10 page and should contain the following:
1. A cover sheet which includes your name (name followed by surname) and your student id followed by the name of your project group and your tutor's name. This is compulsory. Number the pages of your report and also include your name and ID in the footer of each page.
2. The pseudo-code OR flowchart for the problem of your choice, but not both.
3. Your source code (included as text in the report).
4. A simple explanation on how you conducted the testing your program (input data you have used to test whether your program delivers expected outputs, results, or finch behaviour), any errors you have identified during the testing and whether you fixed them.
5. A short statement (maximum 250 words) providing a list of the functionalities that your program does NOT cover. In other words, you should specify parts of the specification that you were not able to implement, if any.
Please note that 10 pages is the maximum and not a requirement for your documentation report, so, do not try to pad out your report needlessly as you may be penalised for the content that is unnecessary or is repetitive. Remember that Blackboard Learn can be very busy close to a deadline so you are advised not to leave submission to the last minute. Even if you are one minute late you still will have missed the deadline. You are also responsible for checking that you have uploaded the correct version of your submission. Once the deadline has passed the submission cannot be changed. However, you may change your submission ONCE prior to the deadline, therefore, a sensible strategy would be to upload your submission several days earlier and then update it later with the latest/final version.
Learning Outcomes
In order to get a pass grade (D- or above) in CS1810, you must meet the learning outcomes below, that is, you must demonstrate ability to:
- Plan, manage and track a non-trivial activity.
- Create and use technical documentation.
- Produce a working computer program as a solution to a given non-trivial problem.
- Develop skills in the use of high-level object-oriented languages.