Section_1
- Describe why an application developer might choose to run over TCP rather than UDP.
- Suppose host A is sending host B a large file over a TCP connection. If the acknowledge number for a segment of this connection is y, then the acknowledge number for the subsequent segment will necessarily be y+ 1. Is this true or false? Why?
- Suppose 5 TCP connections are present over some bottleneck link of rate X bps. All connections have a huge file to send (in the same direction over the bottleneck link). The transmissions of the files start at the same time. What is the transmission rate that TCP would like to give to each of the connections?
- How to identify a UDP socket? How to identify a TCP socket? Are these data fields same? Why?
- UDP and TCP use 1's complement for their checksums. Suppose you have the following three 8-bit words: 01010100, 01111000, 11001101. What is the 1's complement of the sum of these words? Show all work. Why UDP takes the 1's complement of the sum, that is, why not just use the sum?
- Suppose Client A initiates a Telnet session with server S. Provide possible source and destination port numbers for:
a. The segment sent from S to A.
b. The segment sent from A to S.
Compare two pipelining protocols shown - go-back-N and selective Repeat.
In our textbook, protocol rdt 3.0 shows a data transfer protocols that uses only acknowledges. As an alternative, consider a reliable data transfer protocol that uses negative acknowledgements. Suppose the sender sends data only infrequently. Will a NAK-only protocol be preferable to protocol that uses ACKs? Why? Suppose the sender has a lot of data to send and the end-to-end connection experiences few losses. In the second case, would a NAK-only protocol be preferable to a protocol that uses ACKs? Why?
Let us assume that the roundtrip delay between sender and receiver is constant and known to the sender. Would a timer still be necessary in protocol rdt 3.0, assuming that packets can be lost? Please describe.
Section_2: Briefly discuss the basic mechanisms adopted by TCP congestion control.
Describe two major network-layer functions in a datagram network.
Describe how packet loss can occur at input and outputs of a router. Is it possible to eliminate packet loss at these ports? If so, how? If not, please describe.
Suppose an application generates chunks of 200 bytes of data every 20 msec, and each chunk gets encapsulated in a TCP segment and then an IP datagram. What percentage of each datagram will be overhead, and what percentage will be application data?
Consider a datagram network using 8-bit host addresses. Suppose a router uses longest prefix matching and has the following forwarding table:
Prefix Match
|
Interface
|
11
|
0
|
110
|
1
|
otherwise
|
2
|
For each of the 3 interfaces, give the associated range of destination host addresses and the number of addresses in the range.
Consider the following network. With the indicated link costs, use Dijkstra'sshortestpath algorithm to compute the shortest path from x to all network nodes. Show how the algorithm works by computing a table similar to the textbook ex. In cases when several candidate nodes have the same minimal costs, choose a node according to its alphabetical order.
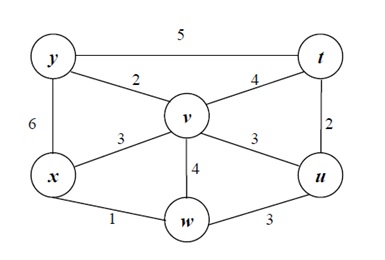
4.6 Consider the count-to-infinity problem in the distance vector routing. Will the problem occur if we decrease the cost of a link? Why?
4.7 IPv6 adopts a fixed-length 40 byte IP header. What is the major advantage of this approach compared to that in IPv4?
4.8 Suppose an ISP owns the block of addresses of the form 200.200.128.0/19. Suppose it wants to create four subnets from this block, with each block having the same number of IP addresses. What are the prefixes (of form a.b.c.d/x) for the four subnets?
Why are different inter-AS and intra-AS protocols used in the Internet?
Part 3. Practical assignment [20 points]
For this assignment, you can choose to use either Java or Python.
Please submit the following items in a ZIP file.
1) Source code;
2) Instructions on how to install and run your program;
3) A brief design document describeing your solution.
Note: I shall not provide remedial help concerning coding problems that you might have. Students are responsible for the setup of their own coding environment. Each student is also expected to debug their code. In addition most SMTP servers (e.g., NSU's email server at nsusmtp.nova.edu) require authentication before sending messages. You can either hard-code the email account's authentication information into the source code, or create a dummy SMTP server to test your program.
Sending Email with Java
Java provides an API for interacting with the Internet mail system, which is called JavaMail. However, we will not be using this API, because it hides the details of SMTP and socket programming. Instead, you should prepare a simple Java program thatestablishes a TCP connection with a mail server through the socket interface, and sends an email message. You can place all of your code into the main method of a class called EmailAgent. Run your program with the following simple command:
javaEmailAgent
This means you will include in your code the details of the particular email message you are trying to send.
Here is a skeleton of the code you'll need to prepare:
import java.io.*;
import java.net.*;
public class EmailAgent
{
public static void main(String[] args) throws Exception
{
// Establish a TCP connection with the mail server.
// Create a BufferedReader to read a line at a time.
InputStream is = socket.getInputStream();
InputStreamReaderisr = new InputStreamReader(is);
BufferedReaderbr = new BufferedReader(isr);
// Read greeting from the server.
String response = br.readLine();
System.out.println(response);
if (!response.startsWith("220")) {
throw new Exception("220 reply not received from server.");
}
// Get a reference to the socket's output stream.
OutputStreamos = socket.getOutputStream();
// Send HELO command and get server response.
String command = "HELO alice\r\n";
System.out.print(command);
os.prepare(command.getBytes("US-ASCII"));
response = br.readLine();
System.out.println(response);
if (!response.startsWith("250")) {
throw new Exception("250 reply not received from server.");
}
// Send MAIL FROM command.
// Send RCPT TO command.
// Send DATA command.
// Send message data.
// End with line with a single period.
// Send QUIT command.
}
}
For this assignment, you are required to use command-line-based Java and should not rely on any features provided by IDE such as NetBeans, Eclipse, etc. In your submission please send me a stand-alone document named "EmailAgent.java". No executables should be submitted. No graphical interface should be used by your program.
Sending Email with Python
You can implement your email client using Python. You should prepare a simple Python program that follow a step-by-step process to establish a TCP connection with a mail server through sockets, and send an email message. The other requirements are very similar to those by choosing Java. I am attaching a skeleton of the Python code below. Again Python 2 is recommended for this assignment.
from socket import *
# Message to send
msg = '\r\nHello World'
endmsg = '\r\n.\r\n'
# Choose a mail server and call it mailserver
mailserver = 'smtp.nova.edu'
# Create socket called clientSocket and establish a TCP connection with mailserver
clientSocket = socket(AF_INET, SOCK_STREAM)
# Port number may change according to the mail server
clientSocket.connect((mailserver, 587))
recv = clientSocket.recv(1024)
printrecv
ifrecv[:3] != '220':
print '220 reply not received from server.'
# Send HELO command and print server response.
heloCommand = 'HELO gmail.com\r\n'
clientSocket.send(heloCommand)
recv1 = clientSocket.recv(1024)
print recv1
if recv1[:3] != '250':
print '250 reply not received from server.'
# Send MAIL FROM command and print server response.
# Send RCPT TO command and print server response.
# Send DATA command and print server response.
# Send message data.
# Message ends with a single period.
# Send QUIT command and get server response.