Data Structures Assignment: InOrder, PreOrder, and PostOrder Traversal in a Binary Search Tree (BST)
In this assignment, you will implement inorder, preorder, and postorder traversal functions on a binary search tree.
public:
void inOrder(void(*func)(const T &)) const;
void preOrder(void(*func)(const T &)) const;
void postOrder(void(*func)(const T &)) const;
private:
void inOrder(void(*func)(const T &), bst_node* node) const;
void preOrder(void(*func)(const T &), bst_node* node) const;
void postOrder(void(*func)(const T &), bst_node* node) const;
Each of these traversal methods matches a public method that takes a function pointer as a parameter with an overloaded private method that takes a function pointer and a binary-search-tree node pointer as parameters. The private method is recursive. The reason the matching of public and private functions is necessary is that the private function takes a node pointer in the second parameter position; node pointers are private to the binary search tree.
Please download the starter files for this assignment from the Files tab (Assignment8.zip). Do not alter the class definition or driver code in any way. Programs that crash are subject to a 50% penalty. Please submit the class header file only ("bst.h"). PLEASE NOTE: You may not use any Standard Template Library (STL) classes for this assignment; use code provided by the instructor only.
Here is an example: suppose you have the following BST:
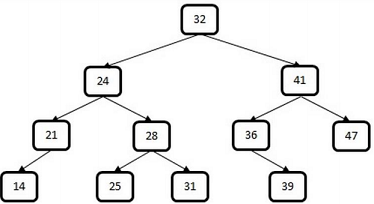
BST.inOrder(); yields 14->21->24->25->28->31->32->36->39->41->47
BST.preOrder(); yields 32->24->21->14->28->25->31->41->36->39->47
BST.postOrder(); yields 14->21->25->31->28->24->39->36->47->41->32
Attachment:- Assignment Files.rar